Boost Your Laravel Performance with Eager Loading Techniques
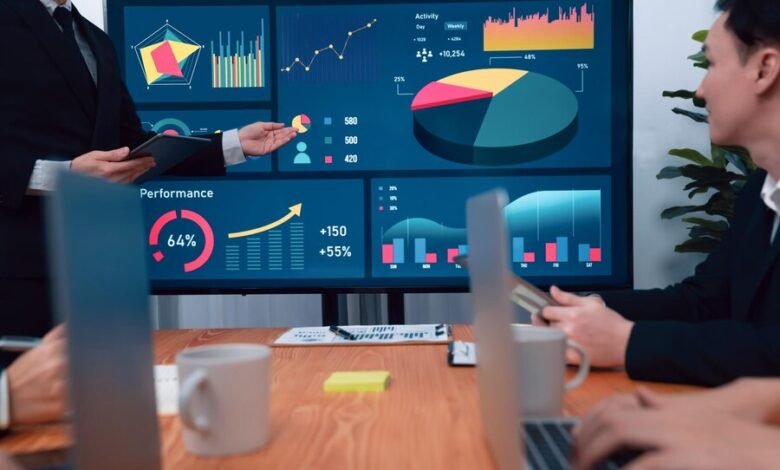
Introduction to Eager Loading and its Importance in Laravel Eloquent
Ever felt like your Laravel application was running slower than it should be? You might not realize it, but how you load your data could be the culprit. Eager loading in Laravel Eloquent is here to save the day. In this blog post, we’ll explore why eager loading is so important and how it can help optimize your application’s performance.
Laravel developers often face performance issues when dealing with large datasets. Eager loading offers a way to mitigate these problems by reducing the number of queries executed. By the end of this post, you’ll have a solid understanding of what eager loading is, why it’s beneficial, and how you can implement it in your Laravel projects.
Let’s jump in and uncover the secrets to speeding up your Laravel applications!
Understanding the Difference Between Lazy Loading and Eager Loading
Before we get into the nitty-gritty of eager loading, it’s crucial to understand the difference between lazy loading and eager loading.
What is Lazy Loading?
Lazy loading is the default behavior in Laravel Eloquent. When you query a model, related models are not retrieved until you explicitly request them. This can lead to what’s known as the “N+1 query problem,” where N represents the number of related records. Essentially, for each record, an additional query is executed to fetch its related data.
For example, if you have 100 users and each user has one profile, lazy loading would execute 101 queries—one for the users and 100 for their profiles.
What is Eager Loading?
Eager loading, on the other hand, retrieves all the necessary related models in a single query. This approach minimizes the number of queries executed, thus enhancing performance. In the same example, eager loading would only execute two queries—one for the users and one for their profiles.
Why Choose Eager Loading?
Choosing eager loading over lazy loading can significantly reduce database queries, improving the speed and efficiency of your application. It’s particularly useful when dealing with large datasets or complex relationships.
Step-by-Step Guide to Implementing Eager Loading in Laravel Eloquent
Now that you understand the basics, let’s walk through how to implement eager loading in your Laravel applications.
Step 1: Basic Eager Loading
To start, you’ll need to modify your existing queries to include eager loading. This is done using the `with` method in your Eloquent queries.
Example:
$users = User::with(‘profile’)->get();
In this example, we are loading users along with their profiles in a single query.
Step 2: Nested Eager Loading
What if you need to load nested relationships? Laravel makes this easy as well.
Example:
$users = User::with(‘profile.address’)->get();
Here, we are loading users, their profiles, and the addresses associated with those profiles in a single query.
Step 3: Conditional Eager Loading
Sometimes, you might want to load related models based on certain conditions. Laravel allows for conditional eager loading.
Example:
$users = User::with([‘profile’ => function ($query) {
$query->where(‘status’, ‘active’);
}])->get();
In this example, only active profiles are loaded along with the users.
Real-World Examples of Eager Loading for Optimal Performance
Understanding theory is one thing, but seeing how it applies in real-world scenarios is incredibly useful. Let’s look at some practical examples.
Example 1: E-commerce Application
Imagine an e-commerce application where you need to display products along with their categories and reviews. Using eager loading, you can do this efficiently.
Example:
$products = Product::with([‘category’, ‘reviews’])->get();
This query retrieves all products, their categories, and reviews in just three queries, regardless of the number of products.
Example 2: Social Media Platform
For a social media platform displaying user posts along with comments and likes, eager loading can optimize performance.
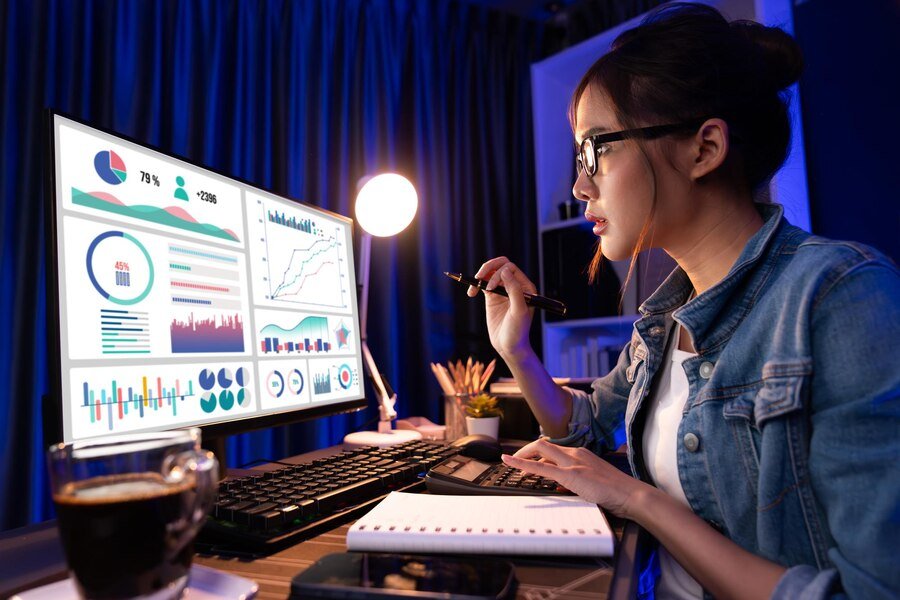
Example:
$posts = Post::with([‘comments’, ‘likes’])->get();
This query fetches posts and their associated comments and likes in minimal queries, speeding up page load times.
Example 3: Educational Portal
In an educational portal, you might need to display students along with their enrolled courses and grades.
Example:
$students = Student::with([‘courses’, ‘grades’])->get();
By using eager loading, you can retrieve all necessary data in a few queries, making your application more efficient.
Best Practices and Common Pitfalls to Avoid
While eager loading is a powerful tool, there are best practices and pitfalls to be aware of.
Best Practices
- Use Eager Loading Wisely: Only use eager loading when necessary. Loading unnecessary relationships can lead to increased memory usage.
- Combine with Pagination: When dealing with large datasets, combine eager loading with pagination to avoid loading too much data at once.
- Monitor Query Performance: Regularly monitor your application’s query performance to ensure that eager loading improves efficiency.
Common Pitfalls
- Overloading Relationships: Loading too many relationships at once can negate the benefits of eager loading. Be selective about what you load.
- Ignoring Recursive Relationships: Be cautious with recursive relationships, as they can lead to performance issues. Use them sparingly.
- Neglecting Indexes: Ensure your database tables are properly indexed to allow for efficient querying, even with eager loading.
Benefits of Eager Loading for Web Developers
Eager loading offers numerous benefits that make it an essential technique for web developers.
Enhanced Performance
By reducing the number of queries executed, eager loading enhances the overall performance of your application, resulting in faster load times and a better user experience.
Simplified Code
Eager loading simplifies your code by reducing the need to manually fetch related models. This leads to cleaner and more maintainable codebases.
Improved Scalability
Applications using eager loading are better equipped to handle large datasets and complex relationships, making them more scalable.
Conclusion
Leveraging eager loading in Laravel Eloquent can significantly optimize your application’s performance. By understanding the difference between lazy loading and eager loading, implementing the latter where necessary, and following best practices, you can create efficient, scalable applications.
If you’re looking to further refine your Laravel skills or need assistance with complex implementations, consider reaching out to experts in the field. Remember, the key to mastering eager loading lies in practice and continuous learning.
Ready to take your Laravel applications to the next level? Start implementing eager loading today and experience the difference it makes!